Application Routing¶
The router lets you define routes that triggers events you can handle by listeners. A route is basically a path which is matched against request.
- Powered by Zend Router
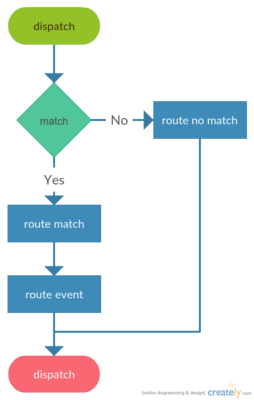
Explanation:
1) Matching a route against request.
- Request made to your application contains a path to the “resource” the client is requesting.
- That request path is matched against available routes until one matches if any.
2) Emitting no route match event.
- When no route was matched, the no route event is emitted to which you can bind like following:
use WebinoAppLib\Event\DispatchEvent;
use WebinoAppLib\Event\RouteEvent;
/** @var WebinoAppLib\Application\AbstractApplication $app */
$app->bind(RouteEvent::NO_MATCH, function (DispatchEvent $event) {
// do something...
});
3) Emitting route match event.
- On a route match, the route match event is emitted to which you can bind like following:
use WebinoAppLib\Event\RouteEvent;
/** @var WebinoAppLib\Application\AbstractApplication $app */
$app->bind(RouteEvent::MATCH, function (RouteEvent $event) {
// do something...
});
4) Emitting route event.
- The matched route event is emitted to which you can bind like following:
use WebinoAppLib\Event\RouteEvent;
/** @var WebinoAppLib\Application\AbstractApplication $app */
// via class
$app->bind(MyRoute::class, function (RouteEvent $event) {
// do something...
});
// or via string
$app->bindRoute('myRoute', function (RouteEvent $event) {
// do something...
});
Routing Interface¶
$app->getRouter()¶
Accessing router service.
/** @var \Zend\Mvc\Router\RouteStackInterface $router */
$router = $app->getRouter();
$app->route()¶
Adding routes.
/** @var \WebinoConfigLib\Feature\Route $route */
$route = $app->route(MyRoute::class)->setLiteral('/');
// or via string
$route = $app->route('myRoute')->setLiteral('/my/route/path');
$app->bind()¶
Binding to routes via class.
use WebinoAppLib\Event\RouteEvent;
/** @var \WebinoAppLib\Application\AbstractApplication $app */
$app->bind(MyRoute::class, function (RouteEvent $event) {
// do something...
});
$app->bindRoute()¶
Binding to routes via class or string.
use WebinoAppLib\Event\RouteEvent;
/** @var \WebinoAppLib\Application\AbstractApplication $app */
// via class
$app->bindRoute(MyRoute::class, function (RouteEvent $event) {
// do something...
});
// via string
$app->bindRoute('myRoute', function (RouteEvent $event) {
// do something...
});
$app->url()¶
Generating URLs.
/** @var \WebinoAppLib\Router\UrlInterface $url */
// via class
$url = $app->url(MyRoute::class);
// or via string
$url = $app->url('myRoute');
Generating URLs HTML.
/** @var \WebinoBaseLib\Html\UrlHtmlInterface $urlHtml */
// via class
$urlHtml = $app->url(MyRoute::class)->html('My Route Label');
// or via string
$urlHtml = $app->url('myRoute')->html('My Route Label');
Available route methods:
$route->setLiteral()¶
Exact matching of the URI path. Configuration is solely the path you want to match.
/** @var WebinoConfigLib\Feature\Route $route */
$route->setLiteral('/route/path');
$route->setSegment()¶
Matching any segment of a URI path. Segments are denoted using a colon, followed by alphanumeric characters. If a segment is optional, it should be surrounded by brackets.
/** @var \WebinoConfigLib\Feature\Route $route */
$route->setSegment('/:requiredParam[/:optionalParam]');
Each segment may have constraints associated with it. Each constraint should simply be a regular expression expressing the conditions under which that segment should match.
/** @var \WebinoConfigLib\Feature\Route $route */
$route
->setSegment('/:requiredParam[/:optionalParam]')
->setConstraints([TODO...]);
Also, as you can in other routes, you may provide defaults to use. These are particularly useful when using optional segments.
/** @var \WebinoConfigLib\Feature\Route $route */
$route
->setSegment('/:requiredParam[/:optionalParam]')
->setDefaults(['optionalParam' => 'defaultValue']);
See also
$route->setDefaults()¶
Setting default route parameters.
/** @var \WebinoConfigLib\Feature\Route $route */
$route->setDefaults(['optionalParam' => 'defaultValue']);
$route->setConstraints()¶
Setting route parameters constraints.
TODO…..
/** @var \WebinoConfigLib\Feature\Route $route */
$route->setConstraints(['optionalParam' => 'TODO...']);
Routing Config¶
Routing Events¶
Following events are emitted during application routing.
RouteEvent::MATCH¶
Route was matched.
This event is emitted at beginning of application dispatch on route match.
use WebinoAppLib\Event\RouteEvent;
$app->bind(RouteEvent::MATCH, function (RouteEvent $event) {});
RouteEvent::NO_MATCH¶
Can’t match a route.
This event is emitted at beginning of application dispatch when route can’t be matched.
use WebinoAppLib\Event\DispatchEvent;
use WebinoAppLib\Event\RouteEvent;
$app->bind(RouteEvent::NO_MATCH, function (DispatchEvent $event) {});
Route Event Interface¶
$event->getParam()¶
Accessing route parameters.
/** @var \WebinoAppLib\Event\RouteEvent $event */
$myParam = $event->getParam('myParam');
$event->getRouteMatch()¶
Obtaining matched route object.
/** @var \WebinoAppLib\Event\RouteEvent $event */
/** @var \Zend\Mvc\Router\Http\RouteMatch $routeMatch */
$routeMatch = $event->getRouteMatch();